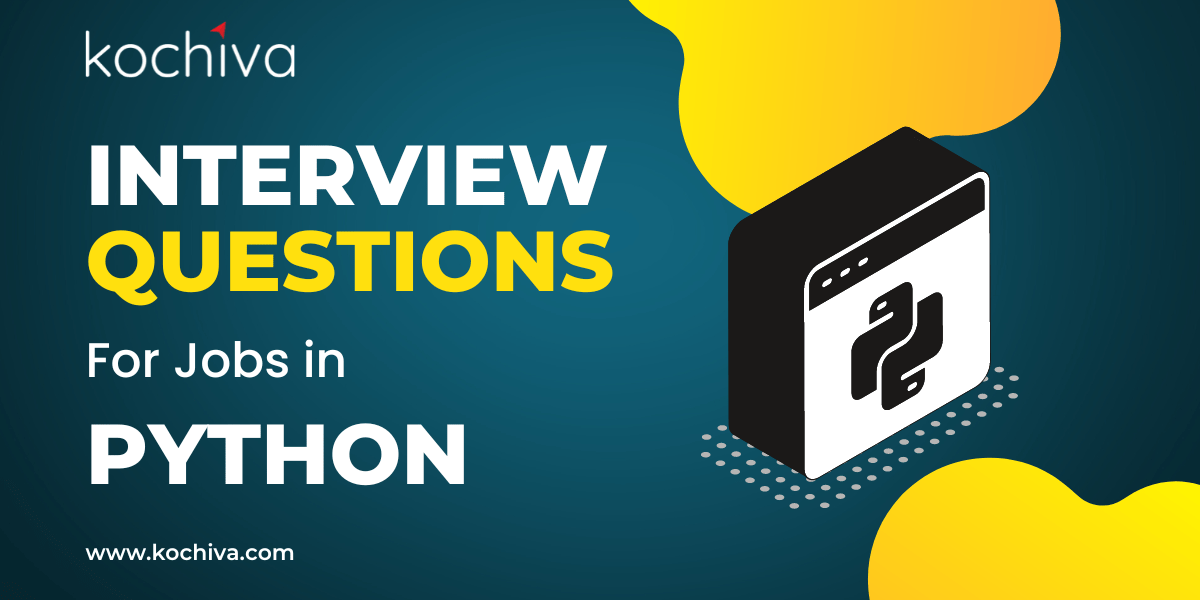
In this blog, let us understand the top Python interview questions to crack any interview. But before that let’s understand, What Python is.
We use Python in coding, software development, machine learning, DevOps, and more. It allows readable syntax and facilitates test-driven application development. In addition, it is platform-friendly and functions on various operating systems like Windows, Linux, or ioS.
This blog provides you with the most frequently asked Python interview questions for freshers. So, let us begin the series of questions:
Top 15 Python Interview Questions and Answers for Freshers in 2024
Q1. What is Python?
Python is a high-level interpreted language. It is a general-purpose programming language that allows it to function with multiple applications when embedded with many tools and libraries.
Python is highly compatible with different platforms such as Windows, Mac, Linux, Raspberry Pi, etc. Furthermore, python supports Objects, Modules, Threads, and automatic memory management that help to model real problems and build applications to resolve these problems.
Some of its advantages are:
- Python provides a simple easy-to-use and learns syntax that enhances readability. The language provides code reusability by encouraging a modular approach.
- In application development, python is highly suitable due to its high-level data structures using the concepts of dynamic binding and typing.
- Furthermore, Python is a productive language. Its simplicity to solve real-world problems saves time and cost making it highly usable.
- Python is highly portable as it can provide the feature of “Write once and run anywhere”.
Q2. What are local, global, and Non-local variables in Python?
Users declare Local variables inside the body of a function i.e. they are in the local scope.
For Example,
def function():
y=”python”
print(y)
function()
Users use Non Local variables in python in nested functions where there is undefined local scope. This points out that the variable neither occurs in the local nor global scope.
The users use the nonlocal keyword for defining nonlocal variables.
For example,
Nonlocal x;
The users declare Global variables in python outside of the function. One can access a global variable either outside or inside of a function.
For example,
y = “python”
def function():
print(y)
function()
print(y)
Q3. What do you mean by PEP 8? Enhance its importance?
PEP signifies Python Enhancement proposal.
It is a document providing guidelines and information that describes the features and the processes to write python code. Documented by Barry Warsaw, Nick Coghlan, and Guido van Rossum in 2001, it focuses on improving the consistency and readability of Python code. This document provides the style guidelines for the code thus making it easier to refer to and analyze. PEP8 is also very suitable for development jobs.
Q4. What are descriptors in Python?
Python V2.2 introduced the Descriptors. A Descriptor is a system behind methods, properties, static methods, class methods, and super().
Also, they allow adding managed attributes to the objects. The methods used to define a descriptor are __getters__, __settters__ and __delete__. Getters and setters are functions in Python that a user uses to adjust attribute values without separate processing. Descriptors use the Python internals by implementing properties and methods. The desciptor is invoked by the method __getattribute__().
Q5. Differentiate between python packages and Python modules?
- A Python Package holds the __init__.py file for user-oriented code. But Modules in runtime may not hold such files for any codes that are user-specific.
- A Package modifies the user-interpreted code in a manner that it queues to function at the runtime only whereas a Module consists of a file that contains python code for a user-specific code in runtime.
Q6. State the purpose of self in python.
Self is a variable that signifies the instance of the class. SELF helps in accessing the variables, methods, and attributes of a defined class in Python.
In other languages using an object-oriented approach, It is passed as a hidden parameter to the methods that are defined by an object. However, in python, it is passed and declared explicitly. It is the first object that is created in the instance of a class with automatic parameters. Different individual objects have separate instances.
Q7. Explain Unit tests in python.
Unit Tests in Python is a unit testing framework allowing separate testing of different components of the software. Unit Tests are different segments of code written to test other pieces of code.
There are two ways of testing:
Manual Testing
The testing is done without following a plan. It involves entering various inputs and checking for the expected outputs on a hit-and-trial basis. This is the most popular way used in testing but is very time-consuming.
Automated Testing
The other type of testing, i.e., Automated testing incorporates code execution according to a systematic code plan. It involves running parts of the code that are decided in a defined order.
Q8. How to create a copy of an object?
In Python = operator is used to creating a copy of an object. Now, think that it creates a new object, but it doesn’t. This only creates a new variable sharing the reference of the original object.
Deep Copy
The copy. deepcopy() function is used to obtain fully independent copies also referred to as deep copies of an object. Deep copies are iterative copies of an interior object.
Shallow Copy
copy. copy() function provides shallow copies of an object. These comprise copies of only the outer container of the objects.
Q9. Difference between Python lists and arrays?
Python Lists –
- Firstly, A list in Python is used to collect items that comprise elements of multiple data types.
- Secondly, The list cannot manage arithmetic operations.
- Also, It comprises elements belonging to the different data types.
- Regarding flexibility, the list is perfect as it allows easy data modification.
- It consumes a more significant memory.
- In a list, the complete list can be accessed without any specific looping.
- Moreover, It favors a shorter sequence of data.
Python Array –
- Firstly, An array serves as an important component that bundles several items of similar built-in data types in Python.
- An array can manage arithmetic operations.
- It incorporates elements of the same data type.
- In terms of flexibility, the array is not suitable as it is not suitable for easy modification of data.
- It consumes less memory than a list.
- In an array, a loop is mandatory to access the array’s components.
- Finally, In an array a loop is mandatory to access the array’s components.
Q 10. How can a Python Script be executable on Unix?
#!/usr/bin/env python must be used at the script’s beginning.
Q11. How do you differentiate between range and xrange? Describe its evolution over time.
The range() and xrange() are two functions in Python that are used to iterate a certain number of times in for loops in Python.
Range returns a list of python objects whereas xrange yields an xrange object. Although both of them provide the same functionalities.
Unlike range which generates a static list during runtime at runtime, xrange uses the method of yielding with large generator objects.
Q12 What does the None value signify in Python?
Users use the “None” value to portray no value or empty value. A function that doesn’t return anything explicitly is assumed to return None. “None” is not similar to 0, False, or an empty String.
Q13. What do you mean by slicing in python?
Slicing means dividing into parts. It is an object that specifies how to slice a sequence.
Python Slice() Function.
The syntax is as : [start: stop: step]
Firstly, Start signifies the initial index when the slicing has to take place.
Stop, signifies the finishing or ending index which marks when slicing stops.
And, Step defines the number of steps that are to be jumped.
Q14.What do you understand by the global, protected, and private scope attributes in Python?
- Another name for Global variables is the global scope. These are the public variables. Users use the Global keyword to put the global scope variable inside a function.
- Private scope attributes are the variables that a user can access only within the scope. One cannot refer from outside directly. Users use two underscores as prefixes for identification.
For example
__data
- Protective attributes are the attributes that a user can access or modify from outside. Users put two underscores as prefixes for their identification.
For example
_data
Q 15. Explain the “with” statement in python.
Programmers extensively use With statement for exceptional handling in python. It ensures much cleaner and more readable code. It helps in the simplification of common resource management such as file streams.
For reference, the following block of code portrays file handling both with the usage of the “with” statement and without it.
# Without using with statement
filepath=open(“the path of the file”,’w)
filepath.write(‘’Python Interview Questions”)
filepath.close()
#Using with statement
With open(“the path of file”,’w’) as filepath:
filepath.write(‘’Python Interview Questions”)
We do not need to call filepath.close() when we apply with statement. The with statement ensures the proper acquisition of resources.
Conclusion
Lastly, in this article, we have covered the most commonly asked interview questions for Python developers. To prepare Python Interview regular practice of these important questions can help you crack any Python Interview.
Over the years, Python has become one of the popular languages among the developer community due to its ability and simplicity to support powerful computations.
Furthermore, if you are a fresher then the interviewer will ask you basic questions rather than complex ones, so try to make your basic concepts very clear. Moreover, look for best Python Training Institute in India and begin your preparations.
Secondly, the thing that matters the most is that whatever you answer, you must answer with confidence. So, just be confident during your interviews.
Moreover, the perks of being a python developer are quite impressive. So, to start your Python journey, get yourself an intensive course. Kochiva provides excellent training in Python and other IT courses.
In addition, you get to have real-time experience learning Python and working on real projects with professionals. Click on Kochiva’s Website and get register for the Python Course to crack any interview.
Keep practicing ! and get closer to your dreams.
In this blog, let us understand the top Python interview questions to crack any interview. But before that let’s understand, What Python is.
We use Python in coding, software development, machine learning, DevOps, and more. It allows readable syntax and facilitates test-driven application development. In addition, it is platform-friendly and functions on various operating systems like Windows, Linux, or ioS.
This blog provides you with the most frequently asked Python interview questions for freshers. So, let us begin the series of questions:
Top 15 Python Interview Questions and Answers for Freshers in 2024
Q1. What is Python?
Python is a high-level interpreted language. It is a general-purpose programming language that allows it to function with multiple applications when embedded with many tools and libraries.
Python is highly compatible with different platforms such as Windows, Mac, Linux, Raspberry Pi, etc. Furthermore, python supports Objects, Modules, Threads, and automatic memory management that help to model real problems and build applications to resolve these problems.
Some of its advantages are:
Q2. What are local, global, and Non-local variables in Python?
Users declare Local variables inside the body of a function i.e. they are in the local scope.
For Example,
def function():
y=”python”
print(y)
function()
Users use Non Local variables in python in nested functions where there is undefined local scope. This points out that the variable neither occurs in the local nor global scope.
The users use the nonlocal keyword for defining nonlocal variables.
For example,
Nonlocal x;
The users declare Global variables in python outside of the function. One can access a global variable either outside or inside of a function.
For example,
y = “python”
def function():
print(y)
function()
print(y)
Q3. What do you mean by PEP 8? Enhance its importance?
PEP signifies Python Enhancement proposal.
It is a document providing guidelines and information that describes the features and the processes to write python code. Documented by Barry Warsaw, Nick Coghlan, and Guido van Rossum in 2001, it focuses on improving the consistency and readability of Python code. This document provides the style guidelines for the code thus making it easier to refer to and analyze. PEP8 is also very suitable for development jobs.
Q4. What are descriptors in Python?
Python V2.2 introduced the Descriptors. A Descriptor is a system behind methods, properties, static methods, class methods, and super().
Also, they allow adding managed attributes to the objects. The methods used to define a descriptor are __getters__, __settters__ and __delete__. Getters and setters are functions in Python that a user uses to adjust attribute values without separate processing. Descriptors use the Python internals by implementing properties and methods. The desciptor is invoked by the method __getattribute__().
Q5. Differentiate between python packages and Python modules?
Q6. State the purpose of self in python.
Self is a variable that signifies the instance of the class. SELF helps in accessing the variables, methods, and attributes of a defined class in Python.
In other languages using an object-oriented approach, It is passed as a hidden parameter to the methods that are defined by an object. However, in python, it is passed and declared explicitly. It is the first object that is created in the instance of a class with automatic parameters. Different individual objects have separate instances.
Q7. Explain Unit tests in python.
Unit Tests in Python is a unit testing framework allowing separate testing of different components of the software. Unit Tests are different segments of code written to test other pieces of code.
There are two ways of testing:
Manual Testing
The testing is done without following a plan. It involves entering various inputs and checking for the expected outputs on a hit-and-trial basis. This is the most popular way used in testing but is very time-consuming.
Automated Testing
The other type of testing, i.e., Automated testing incorporates code execution according to a systematic code plan. It involves running parts of the code that are decided in a defined order.
Q8. How to create a copy of an object?
In Python = operator is used to creating a copy of an object. Now, think that it creates a new object, but it doesn’t. This only creates a new variable sharing the reference of the original object.
Deep Copy
The copy. deepcopy() function is used to obtain fully independent copies also referred to as deep copies of an object. Deep copies are iterative copies of an interior object.
Shallow Copy
copy. copy() function provides shallow copies of an object. These comprise copies of only the outer container of the objects.
Q9. Difference between Python lists and arrays?
Python Lists –
Python Array –
Q 10. How can a Python Script be executable on Unix?
#!/usr/bin/env python must be used at the script’s beginning.
Q11. How do you differentiate between range and xrange? Describe its evolution over time.
The range() and xrange() are two functions in Python that are used to iterate a certain number of times in for loops in Python.
Range returns a list of python objects whereas xrange yields an xrange object. Although both of them provide the same functionalities.
Unlike range which generates a static list during runtime at runtime, xrange uses the method of yielding with large generator objects.
Q12 What does the None value signify in Python?
Users use the “None” value to portray no value or empty value. A function that doesn’t return anything explicitly is assumed to return None. “None” is not similar to 0, False, or an empty String.
Q13. What do you mean by slicing in python?
Slicing means dividing into parts. It is an object that specifies how to slice a sequence.
Python Slice() Function.
The syntax is as : [start: stop: step]
Firstly, Start signifies the initial index when the slicing has to take place.
Stop, signifies the finishing or ending index which marks when slicing stops.
And, Step defines the number of steps that are to be jumped.
Q14.What do you understand by the global, protected, and private scope attributes in Python?
For example
__data
For example
_data
Q 15. Explain the “with” statement in python.
Programmers extensively use With statement for exceptional handling in python. It ensures much cleaner and more readable code. It helps in the simplification of common resource management such as file streams.
For reference, the following block of code portrays file handling both with the usage of the “with” statement and without it.
# Without using with statement
filepath=open(“the path of the file”,’w)
filepath.write(‘’Python Interview Questions”)
filepath.close()
#Using with statement
With open(“the path of file”,’w’) as filepath:
filepath.write(‘’Python Interview Questions”)
We do not need to call filepath.close() when we apply with statement. The with statement ensures the proper acquisition of resources.
Conclusion
Lastly, in this article, we have covered the most commonly asked interview questions for Python developers. To prepare Python Interview regular practice of these important questions can help you crack any Python Interview.
Over the years, Python has become one of the popular languages among the developer community due to its ability and simplicity to support powerful computations.
Furthermore, if you are a fresher then the interviewer will ask you basic questions rather than complex ones, so try to make your basic concepts very clear. Moreover, look for best Python Training Institute in India and begin your preparations.
Secondly, the thing that matters the most is that whatever you answer, you must answer with confidence. So, just be confident during your interviews.
Moreover, the perks of being a python developer are quite impressive. So, to start your Python journey, get yourself an intensive course. Kochiva provides excellent training in Python and other IT courses.
In addition, you get to have real-time experience learning Python and working on real projects with professionals. Click on Kochiva’s Website and get register for the Python Course to crack any interview.
Keep practicing ! and get closer to your dreams.
Best Online Spanish Classes in India
Best Online German Classes in India
Best Online French Classes in India
Preparation for Goethe B2 Exam: All You Need to Know
Best Spanish Language Classes in Canberra
Best German Classes in Thane
Request a Call Back
Related Posts
Preparation for Goethe B2 Exam: All You Need to Know
Read MoreBlog Summary: Qualifying for the B2 exam is challenging. Here are the exam pattern, eligibility criteria, and preparation strategy tips with the best books to help you pass the German Goethe B2 exam in one go. If you take the B2 level German exam, you are just one step away from being eligible for German […]
Best Spanish Language Classes in Canberra
Read MoreCanberra, the capital city of Australia, offers a unique opportunity for individuals looking to expand their linguistic skills, particularly in Spanish. The city’s diverse cultural landscape and educational resources make it an ideal location for learning new languages. Spanish, the second most spoken language in the world, opens numerous doors for personal and professional growth. […]
Best German Classes in Thane
Read MoreAre you looking for German classes in Thane? Look no more; you are exactly where you need to be. Thane is a metropolitan city with much more to offer than lakes. It is one of the busiest cities in Maharashtra, and the trend of learning new languages is growing there. Imagine having the upper hand […]
Top 5 French Classes in Udaipur – Online French Classes
Read MoreLearning French in Udaipur is becoming increasingly popular. As the city connects more with the world, it becomes a fantastic place to learn French, mainly if you aim to boost your career in multinational companies, tourism, or diplomatic services. Consequently, more employers are seeking French-speaking professionals and a French classes in Udaipur can give you […]
Online Spanish Classes For Spanish Language Certification
Read MoreOver the past few years, online language courses have become increasingly popular due to their accessibility, flexibility, and user-friendliness. It’s possible to get a quality education with online Spanish courses for Spanish language certification. If you aim to reach a certain level for a job, test, or school application, you’ll likely want to find online […]
Meet Our Conversion Expert